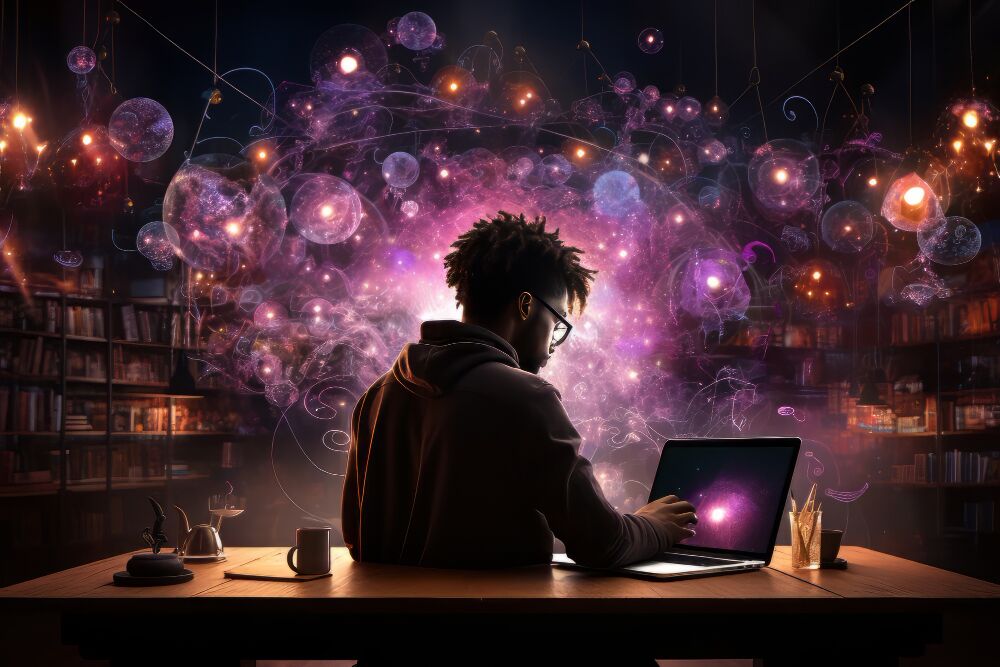
Introduction to Cosmosdb
Azure CosmosDB is a globally distributed, multi-model database service designed to enable you to elastically and independently scale throughput and storage across any number of Azure regions worldwide. It offers turnkey global distribution, elastic scaling of throughput and storage worldwide, single-digit millisecond latencies at the 99th percentile, guaranteed high availability, and automatic, instant, and unlimited scalability.
Definition and overview of Cosmos DB
Azure Cosmos DB is Microsoft’s globally distributed, multi-model database service for operational and analytics workloads. It offers turnkey global distribution, elastic scaling of throughput and storage worldwide, single-digit millisecond latencies at the 99th percentile, guaranteed high availability, and automatic, instant, and unlimited scalability.
Key capabilities of Azure Cosmos DB include:
- Turnkey global distribution
- Elastic scaling of throughput and storage worldwide
- Single-digit millisecond latencies at the 99th percentile
- High availability with SLAs
- Automatic and instant scalability
- Open APIs for many database engines
The role of Cosmos DB in modern app development
Azure CosmosDB plays a critical role in modern application development by enabling developers to elastically scale throughput and storage across any number of geographical regions with a click of a button. Its global distribution and multi-model capabilities make it a great fit for web, mobile, gaming, IoT and many other applications that require seamless scalability and high performance.
Benefits of Azure Cosmos Database
Some of the key benefits of Azure Cosmos DB include:
- Global distribution out of the box
- Automatic sharding
- Elastic scalability of storage and throughput
- Guaranteed low latency
- Five well-defined consistency models
- Comprehensive service level agreements (SLAs)
- Multi-model API support
- Fully managed
- Enterprise-grade security
Disadvantages of Azure Cosmos DB – Pricing, Anyone?
While Azure CosmosDB offers many benefits, there are some limitations to consider:
- Complex pricing model based on provisioned throughput
- Can be more expensive than other databases for light workloads
- Limited query support compared to traditional RDBMS
- Less flexibility for customization compared to self-managed NoSQL databases
Careful capacity planning and cost optimization is necessary to run cost-effective production workloads on Cosmos DB.
An Introduction to Azure Cosmos Database
Introduction to Azure Cosmos Database data architecture
The Azure Cosmos DB database service is designed from the ground up to natively support multiple data models:
- Key-value
- Column-family
- Document
- Graph
The database engine itself is schema-agnostic, meaning it does not rely on any schema or index definitions. Azure Cosmos DB automatically indexes all data without requiring any schema or secondary indexes from the developer.
Cosmos DB data model support
Azure Cosmos DB supports multiple APIs, allowing you to access Azure Cosmos DB databases with the API of your choice:
- SQL (DocumentDB): Offers JSON documents with SQL querying and JavaScript procedural logic
- MongoDB: The popular document database for modern applications
- Cassandra: The leading NoSQL column-family database
- Gremlin (Graph): A graph database with nodes, edges and properties
- Table: Key-value store with schema
Globally distributed database
Azure Cosmos DB replicates data across any number of Azure regions of your choice, providing low latency access to data anywhere. It also offers transparent multi-homing APIs that allow accessing data from any region.
High availability and low latency
Azure CosmosDB guarantees less than 10 ms reads and under 15 ms writes at the 99th percentile. It also offers industry-leading comprehensive SLAs for throughput, latency, availability and consistency.
Automatic and instant scalability
Azure Cosmos DB enables unlimited, instantaneous elastic scaling of throughput and storage across any number of regions worldwide. You can elastically scale up to meet sudden spikes in traffic.
Enterprise-grade security
Azure Cosmos DB provides enterprise-grade security and compliance capabilities including role-based access control, firewall support, customer-managed keys for encryption, and compliance with SOC, ISO, HIPAA and PCI DSS standards.
API options and their use cases
API for MongoDB-specific limits
While Azure Cosmos DB API for MongoDB provides wire protocol level compatibility for MongoDB workloads, there are some limitations to be aware of:
- Limited support for some aggregation pipeline operators
- Lack of multi-document ACID transactions
- No support for MongoDB Change Streams
Carefully validate any use of unsupported features before migrating MongoDB apps to Cosmos DB.
Setting up an Azure CosmosDB account
Creating an Azure Cosmos DB account
You can create an Azure Cosmos DB account using:
- Azure portal
- Azure CLI
- Azure PowerShell
- ARM templates
Be sure to specify the API type, consistency level, regions, and initial throughput you need.
Accessing CosmosDB using PowerShell libraries
You can manage and access Azure Cosmos DB accounts using Azure PowerShell libraries like Az.CosmosDB
. Key operations include creating and updating databases, containers, items and stored procedures.
Here is sample PowerShell code to connect and access Azure Cosmos DB:
Import-Module Az.CosmosDB
$cosmosDbContext = New-AzCosmosDBContext -AccountEndpoint "https://contoso.documents.azure.com" -AccountKey "accountkey"
# Create a new database and container
New-AzCosmosDBSqlDatabase -Context $cosmosDbContext -Name myDatabase -Throughput 400
New-AzCosmosDBSqlContainer -Context $cosmosDbContext -Name myContainer -DatabaseName myDatabase -PartitionKeyKind Hash -PartitionKeyPath "/myPartitionKey" -Throughput 400
# Insert, query and delete items
$document = New-AzCosmosDBSqlDocument @(@{id="1"; category="test"}, @{id="2"; category="test2"})
New-AzCosmosDBSqlDocument -Context $cosmosDbContext -CollectionName myContainer -DocumentBody $document
Get-AzCosmosDBSqlDocument -Context $cosmosDbContext -CollectionName myContainer -Query "SELECT * FROM c WHERE c.category = 'test'"
Remove-AzCosmosDBSqlDocument -Context $cosmosDbContext -CollectionName myContainer -Id "1"
This demonstrates basic CRUD operations from PowerShell. You can also create and execute stored procedures, user defined functions, and triggers programmatically.
CosmosDB and NodeJS
You can access Azure Cosmos DB from Node.js applications using the @azure/cosmos
package:
const CosmosClient = require('@azure/cosmos').CosmosClient
const client = new CosmosClient({ endpoint, key })
// Database operations
const database = client.database(databaseId)
await database.containers.createIfNotExists({ id: containerId })
// Container operations
const container = client.database(databaseId).container(containerId)
const { resources } = await container.items.query('SELECT * from c').fetchAll()
The Azure Cosmos DB Node.js SDK supports all database operations including CRUD, queries, stored procedures and triggers.
SQL query in Cosmos DB using ODBC
Azure Cosmos DB provides an ODBC driver to connect using SQL querying tools:
// Connect via ODBC
string connectionString =
"Driver={Azure Cosmos DB ODBC Driver};" +
"AccountEndpoint=https://your-account.documents.azure.com;"+
"AccountKey=your-account-key;"+
"Database=your-database;"+
"MultiSubnetFailover=true";
SqlConnection conn = new SqlConnection(connectionString);
conn.Open();
SqlCommand cmd = new SqlCommand("SELECT * FROM myContainer", conn);
Make sure to include your account endpoint, key, database and container names in the connection string.
Working with CosmosDB
Importing data with Azure Cosmos DB migration tool
The Azure Cosmos DB migration tool allows importing data from:
- JSON files
- MongoDB
- SQL Server
- Azure Table storage
- Amazon DynamoDB
- CSV files
To use the data migration tool:
- Install it from GitHub
- Connect to source and target databases
- Map source to target containers
- Configure throughput provisioning
- Select data to migrate
- Start and monitor migration
Adding a Composite Index
You can create a composite index in Azure Cosmos DB using the SQL API:
// Composite index
{
"indexingMode": "consistent",
"includedPaths": [
{
"path": "/age/*",
"indexes": [
{
"kind": "Range",
"dataType": "Number"
}
]
},
{
"path": "/name/*",
"indexes": [
{
"kind": "Hash",
"dataType": "String",
"precision": 3
}
]
}
]
}
The path defines the property paths to index, while the indexes array specifies the index kinds per path – hash, range, spatial and composite indexes are possible.
Indexing in Azure Cosmos Database
Azure Cosmos DB is a schema-agnostic database that automatically indexes all properties in documents without requiring any schema or secondary index management.
It offers:
- Automatic indexing of every property
- Tunable consistency levels
- Configurable index policies
- Composite indexes
- Spatial and geospatial support
- Range indexes for sorting and comparisons
Composite indexes allow combining multiple properties to efficiently query documents.
SQL query limits
Azure Cosmos DB has the following limitations for SQL query statements:
- Maximum 2 MB request size
- Maximum 5 seconds execution before timeout
- Maximum 512 MB query response size
Per-request limits apply
In addition to account-level throughput limits, Azure Cosmos DB enforces per-request limits:
- Maximum 2 MB request size
- Maximum 5 seconds execution before timeout
- Maximum 1 MB response size
Per-container limit
There is also a 20 GB size limit per partition key value, to ensure evenly distributed partitions. Design your partition key scheme to avoid hitting this limit.
Azure Cosmos DB free tier account limits
The Azure Cosmos DB free tier has the following limits:
- 400 RU/s provisioned throughput
- 5 GB storage
- Shared performance and scalability
If your needs exceed these free tier limits, you will need to create a standard S1 or higher tier Azure Cosmos account.
Control Planes
Understanding control planes in CosmosDB
The control plane refers to the services and APIs used to manage an Azure Cosmos DB account:
- Azure portal
- Azure Resource Manager
- Azure Cosmos DB SDKs
- Azure CLI and Azure PowerShell
These interfaces allow administering Azure Cosmos DB accounts globally, including operations like adding and removing regions, failover priorities, scaling throughput, adding containers, etc.
Conclusion
Azure Cosmos DB provides a highly scalable, globally distributed database service with multiple APIs and native support for multiple data models. With features like automatic indexing, tunable consistency levels and comprehensive SLAs, CosmosDB is a robust platform for building scalable, high performance modern applications.
Careful planning around partitioning, indexing, queries and throughput provisioning is necessary to build cost-effective, high-scale production applications on Cosmos DB.
You can try Azure Cosmos DB for free without an Azure subscription using the Azure CosmosDB Free Tier. The free tier is a great way to test Cosmos DB capabilities without incurring any costs.
References